Ollama 快速入门:Ollama Python Library
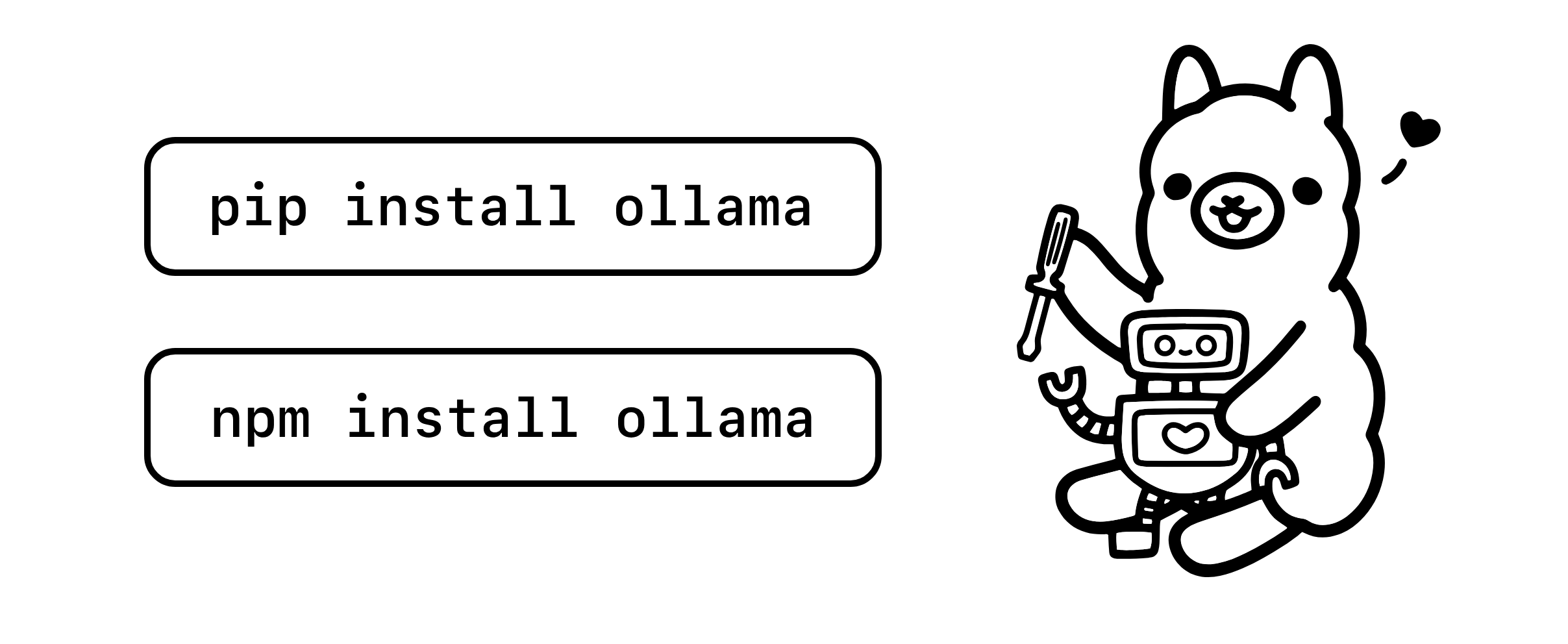
Ollama Python 库提供了将 Python 3.8+ 项目与 Ollama 集成的最简单方法。
先决条件
您需要运行本地 ollama 服务器才能继续。具体操作如下:
运行一个本地 LLM:https://ollama.com/library
- 例子:
ollama run llama3
- 例子:
ollama run llama3:70b
- 例子:
然后:
curl https://ollama.ai/install.sh | sh ollama serve
接下来您可以继续 ollama-python。
安装
pip install ollama
用例
import ollama
response = ollama.chat(model='llama2', messages=[
{
'role': 'user',
'content': 'Why is the sky blue?',
},
])
print(response['message']['content'])
流式响应
可以通过设置 stream=True
、修改函数调用来启用响应流,以返回一个 Python 生成器,其中每个部分都是流中的一个对象。
import ollama
stream = ollama.chat(
model='llama2',
messages=[{'role': 'user', 'content': 'Why is the sky blue?'}],
stream=True,
)
for chunk in stream:
print(chunk['message']['content'], end='', flush=True)
API
Ollama Python 库的 API 是围绕 Ollama REST API 设计的.
Chat
ollama.chat(model='llama2', messages=[{'role': 'user', 'content': 'Why is the sky blue?'}])
Generate
ollama.generate(model='llama2', prompt='Why is the sky blue?')
List
ollama.list()
Show
ollama.show('llama2')
Create
modelfile='''
FROM llama2
SYSTEM You are mario from super mario bros.
'''
ollama.create(model='example', modelfile=modelfile)
Copy
ollama.copy('llama2', 'user/llama2')
Delete
ollama.delete('llama2')
Pull
ollama.pull('llama2')
Push
ollama.push('user/llama2')
Embeddings
ollama.embeddings(model='llama2', prompt='They sky is blue because of rayleigh scattering')
自定义客户端
可以使用以下字段创建自定义客户端:
host
: 要连接的 Ollama 主机timeout
: 请求超时
from ollama import Client
client = Client(host='http://localhost:11434')
response = client.chat(model='llama2', messages=[
{
'role': 'user',
'content': 'Why is the sky blue?',
},
])
异步客户端
import asyncio
from ollama import AsyncClient
async def chat():
message = {'role': 'user', 'content': 'Why is the sky blue?'}
response = await AsyncClient().chat(model='llama2', messages=[message])
asyncio.run(chat())
设置 stream=True
修改函数以返回 Python 异步生成器:
import asyncio
from ollama import AsyncClient
async def chat():
message = {'role': 'user', 'content': 'Why is the sky blue?'}
async for part in await AsyncClient().chat(model='llama2', messages=[message], stream=True):
print(part['message']['content'], end='', flush=True)
asyncio.run(chat())
错误
如果请求返回错误状态或在流式传输过程中检测到错误,则会引发错误。
model = 'does-not-yet-exist'
try:
ollama.chat(model)
except ollama.ResponseError as e:
print('Error:', e.error)
if e.status_code == 404:
ollama.pull(model)
参考资料:
作者:Jeebiz 创建时间:2024-05-12 21:44
最后编辑:Jeebiz 更新时间:2025-04-09 22:19
最后编辑:Jeebiz 更新时间:2025-04-09 22:19